KML Layers
When the general tools of Google Maps are not enough, you can always turn to KML layers (opens new window) in order to "kick it up a notch". Within the context of a KML layer, the options to decorate a map are practically boundless.
Here is a map of the Chicago subway system using a KML layer...
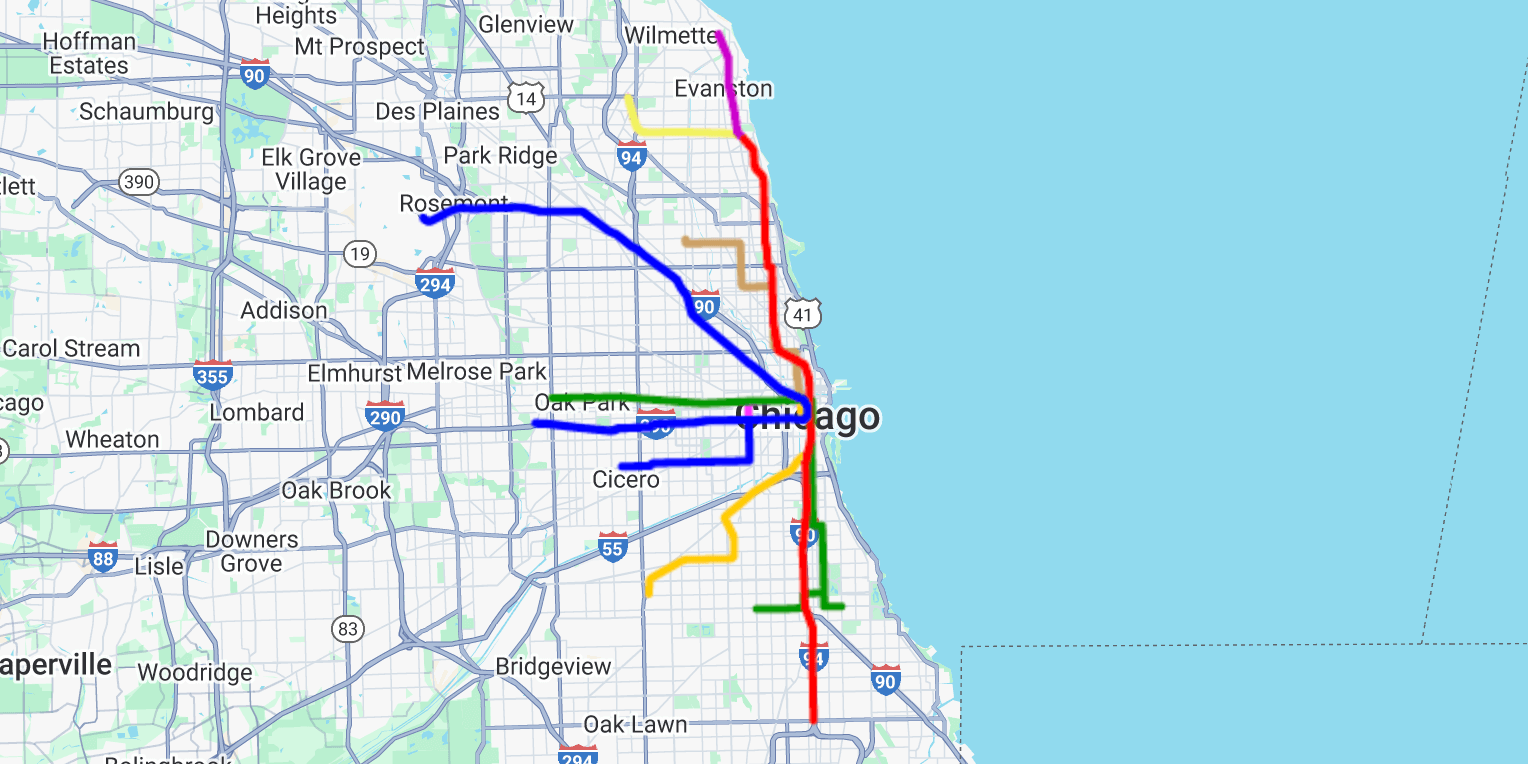
There are a few tools for creating KML layers, here's the official Google resource:
However you go about creating your KML layer, it's easy to apply it to an existing map...
map.kml(url, options);
Practically speaking, your code could look something like this...
// Create a plain dynamic map
const map = googleMaps.map();
// Add a KML layer to the map
map.kml(url);
For more information, take a look at the details of the kml
method. If you intend to further manipulate the KML layers, it will be necessary to provide an id
value.
# The url
parameter
The most important things to note about the url
parameter:
- You must use a complete URL (including the
http(s)://
prefix). - You must use a publicly accessible URL (because Google needs to parse it).
URL must be publicly accessible
When Google loads the map, it needs to internalize and process the KML file. Because of this, it's not possible for Google to parse KML files that are not publically accessible.
If you are testing this feature locally, the KML file may refuse to load (opens new window).
# The options
parameter
All options are optional.
Within the context of kmlLayerOptions
, you can specify any attributes of a KmlLayerOptions
(opens new window) interface. There's no need to specify map
or url
values, as they will be set automatically.
map.kml(url, {
'id': 'my-kml',
'kmlLayerOptions': {
'preserveViewport': true
}
});
Further KML Adjustments
To manipulate a KML layer, you will need to refer to it by its assigned ID. If you plan to manipulate the KML layers, be sure to set the id
option of each KML layer when initially adding it to the map.
# Further manipulating KML layers
If you only need to hide or show each KML layer, there are two convenient universal methods...
// Hide the KML layer
map.hideKml('my-kml');
// Show the KML layer
map.showKml('my-kml');
# Beyond hiding and showing
When you need to do more than hide or show a layer, you can get the raw Google KML layer object (opens new window), and manipulate it further using Google's own API.
This method is only available in JavaScript.
// Get the raw Google KML layer object
const kml = map.getKml('my-kml');