Drawing Circles
Using the Google API, it's possible to draw circles (opens new window) on your dynamic maps.
We've brought this functionality into the plugin. The circles
method is part of the Universal API, so you can use it in any available language...
map.circles(locations, options);
Specify one or more locations, and optionally provide an array of options.
There are only two options
(both optional)...
Option | Type | Description |
---|---|---|
id | string | Reference point for each circle. |
circleOptions | array | Accepts any google.maps.CircleOptions (opens new window) properties. |
# Circle Options
Be aware, most of what you want to control will be nested within the circleOptions
option. In essence, you'll need to go two layers deep to configure each set of circles.
Here is a practical example in Twig...
{# Get all circle locations #}
{% set locations = craft.entries.section('circles').all() %}
{# Note that `circleOptions` are nested within options #}
{% set options = {
'circleOptions': {
'radius': 50000,
'fillColor': '#7A9F35',
'fillOpacity': 0.35,
'strokeColor': '#7A9F35',
'strokeOpacity': 0.8,
'strokeWeight': 2,
}
} %}
{# Show a map with circles #}
{{ googleMaps.map().circles(locations, options).tag() }}
In the example above, all circles will be the exact same size and color.
# Setting a Radius
# The radius must be nested below circleOptions
.
As noted above, be sure that the radius
value is specified within the circleOptions
option.
# The radius must have a value in meters.
The Google Maps API is expecting the radius
to be specified in meters (opens new window).
// Draw a circle with a 5 kilometer radius
const options = {
'circleOptions': {
'radius': 5000
}
};
If you are performing a calculation to determine the radius value dynamically, be sure that it is ultimately returning a value in meters.
# Circles of Different Sizes/Colors
It's possible to add multiple circles that are different sizes and/or colors.
# Simple Example
Create each circle individually by looping through all locations and configuring them one at a time...
{# Initialize a map object #}
{% set map = googleMaps.map() %}
{# Loop over all circles #}
{% for entry in craft.entries.section('circles').all() %}
{# Set the options for each circle individually #}
{% set options = {
'circleOptions': {...}
} %}
{# Add configured circle to the map #}
{% do map.circles(entry, options) %}
{% endfor %}
# Complex Example
Here's one possible way to dynamically manage the size and color of each circle...
{# Set the shared options for all circles #}
{% set baseCircleOptions = {
'fillOpacity': 0.35,
'strokeOpacity': 0.8,
'strokeWeight': 2,
} %}
{# Initialize a map object #}
{% set map = googleMaps.map() %}
{# Loop over all circles #}
{% for entry in craft.entries.section('circles').all() %}
{# Set the options for a single circle #}
{% set options = {
'circleOptions': baseCircleOptions|merge({
'radius': (entry.radius * 1000),
'fillColor': entry.color.getHex(),
'strokeColor': entry.color.getHex(),
})
} %}
{# Add configured circle to the map #}
{% do map.circles(entry, options) %}
{% endfor %}
In the example above, we've also created two new fields:
- Radius - Determines the size of the circle.
- Color - Determines the color of the circle.
These fields will live alongside our Address field, which determines the location of each circle.
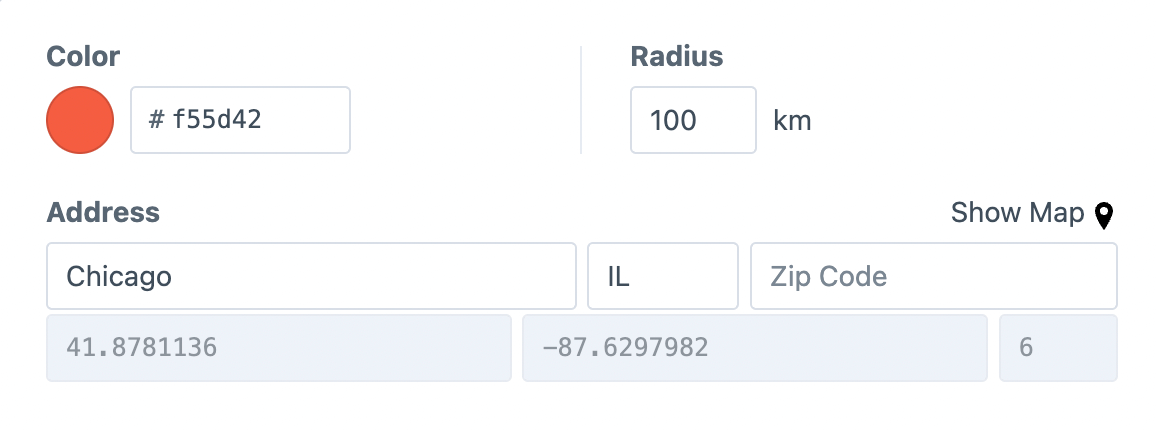
Once you have those fields and Twig snippet in place, you'll be able to generate a map like this...
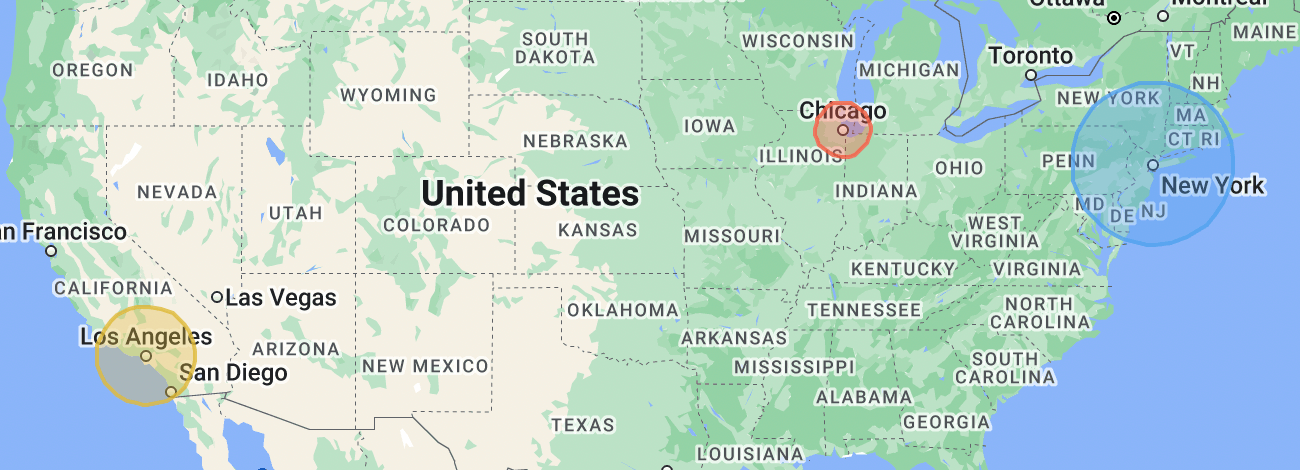
Various Approaches
While the example above uses two additional fields (Radius and Color) to control the circle options, there are many other ways to manage how your circles are displayed. Feel free to adapt this approach to better fit your existing architecture.
# Hiding or Showing a Circle
To dynamically show or hide your circles, you can use hideCircle
and showCircle
...
// Hide a circle
map.hideCircle(circleId);
// Show a circle
map.showCircle(circleId);
The circleId
is a string (or array) which identifies the specific circle(s) that you are targeting.
Format | Type | |
---|---|---|
One circle | '{elementId}-{fieldHandle}' | string |
Multiple circles | An array of circle IDs | array |
All circles | '*' | string |
More Info
For more information, see the Universal API regarding hideCircle
and showCircle
.