Setting Marker Icons
There are two opportunities to set a marker icon:
- When the marker is created (using the
markers
method ormap
method) - After the marker already exists (using the
changeMarker
method)
# Creating Marker Icons
When the map is initially created, or markers are initially placed, you can use the markerOptions
option to control how the marker looks and behaves.
If you've got multiple groups of markers, you can specify different marker options for each batch of markers.
// Get all bars & restaurants
const bars = {'lng': -73.935242, 'lat': 40.730610}; // Coords only in JS
const restaurants = {'lng': -118.243683, 'lat': 34.052235}; // Coords only in JS
// Create a dynamic map (with no markers)
const map = mapbox.map();
// Add all bar markers (blue)
map.markers(bars, {
'markerOptions': {'color': '#197BBD'}
});
// Add all restaurant markers (red)
map.markers(restaurants, {
'markerOptions': {'color': '#BE3100'}
});
// Display map (inject into `#my-map-container`)
map.tag({'parentId': 'my-map-container'});
# Changing Marker Icons
It's also possible to change the options of an existing marker. The changeMarker
method accepts all the same Marker
parameters (opens new window) as the initial map or marker creation.
map.changeMarker(markerId, markerOptions);
Using the changeMarker
method, it's possible to change one or more markers at a time.
// Change one marker
map.changeMarker('33-address', {'color': '#197BBD'});
// Change specified markers
map.changeMarker(['33-address', '42-address'], {'color': '#197BBD'});
// Change all markers
map.changeMarker('*', {'color': '#197BBD'});
Getting the Marker IDs
To see the existing marker IDs (if you didn't manually specify them), do the following:
- Put the site into devMode (opens new window).
- View the JS console while the map is being rendered.
In the JavaScript console, you should see a complete play-by-play of every map component being created. Simply copy & paste the marker ID's you need from there, or take note of the pattern for your own needs.
# Image as Marker Icon
When you need more control than just changing the marker's color, it's possible to use your own <img>
(or other HTML) for custom marker icons.
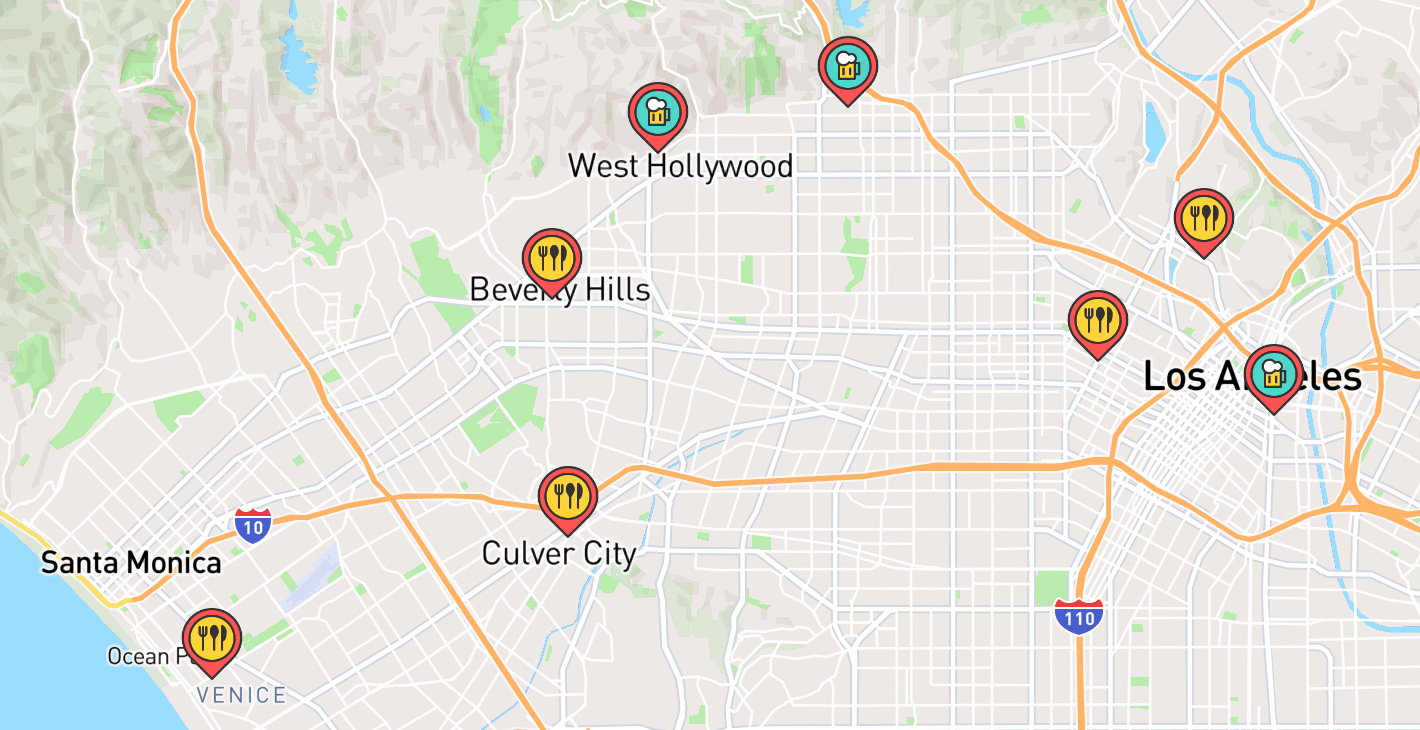
- Start by adding the marker icon element to the DOM...
<div style="display: none">
<img id="custom-marker-icon" src="path/to/icon/image.png">
</div>
Element must exist, but can be hidden
The specified element must exist in the DOM before the map is rendered.
However, you can safely store it within a hidden (display: none
) container.
- When adding
markers
, specify the existing DOM element within themarkerOptions
option.
Use the element
parameter to reference the DOM element by its id
...
const markerOptions = {
'element': '#custom-marker-icon',
'anchor': 'bottom'
}
Anchor
You will likely also need to specify the anchor
parameter (opens new window). By default, Mapbox will try to align the center of your icon with its corresponding coordinates.
# JavaScript Only
If you are working with JavaScript, you can potentially create a DOM element on the fly.
Use JavaScript to create a new dynamic DOM element, then pass in the entire element
.
// Dynamically create an image element
const img = document.createElement('img');
img.src = 'path/to/icon/image.png';
// Use the new element as the marker icon
const markerOptions = {
'element': img,
'anchor': 'bottom'
}